The default template for a Windows application comes with almost no guidance for how developers should do even basic things like logging. Luckily, the Uno Platform wizard provides a simple way to create an application that configures the Microsoft.Extensions for each of the supported platforms. This includes the ability to setup Microsoft.Extensions.Logging with the ability to set minimum logging level and add filters for classes and namespaces. In this post we’re going to configure logging to write to Azure Application Insights.
Let’s create a new application using the Uno Platform Template Wizard – by default this will create a cross platform application that includes all the supported Uno Platform targets but if you only want to target Windows (or a different subset of the platforms) you can adjust this from the Platforms tab.
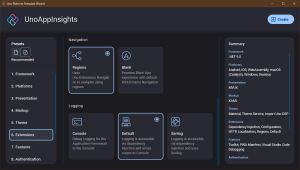
We’re going to go with the Recommended preset, which includes support for Microsoft.Extensions. In the Logging section, we’ll stick with Default for now, but it’s useful to know that you can select the Serilog option if you want to write log information to files.
Ok, next up we need to add a reference to the Microsoft.Extensions.Logging.ApplicationInsights nuget package.
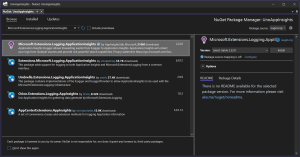
If you don’t already have an Azure Application Insights resource, take the opportunity to create one now via the Azure Portal. Once you’ve created the Application Insights resource, copy the Connection String value for use in the next step.
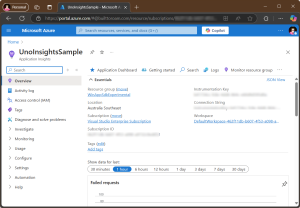
In Solution Explorer, locate the appsettings.json file (or if you only want to set different values for development than for production, locate the appsettings.development.json file) and add a new section, TelemetryConfiguration, with a single node, ConnectionString, which contains the Connection String copied from the Azure portal.
{
... ,
"TelemetryConfiguration": {
"ConnectionString": "InstrumentationKey=XXXXXXXX"
}
}
Next we need to call logBuilder.AddApplicationInsights()
, as part of configuring the application Host. This will setup logging to output to Application Insights.
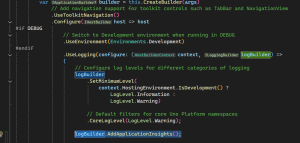
We’ll also configure an instance of the TelemetryClient as a service that can be used to track events.
services.AddSingleton(sp => new TelemetryClient(sp.GetRequiredService>().Value));

And that’s all we need to do to install and configure support for Azure Application Insights in our application.
We can make use of this in two ways. Firstly, when the application calls any of the log methods on ILogger, a trace is added to Application Insights. Alternatively, the application can access the TelemetryClient and use it to track events.
var logger = Host.Services.GetRequiredService<ILogger<App>>();
logger.LogError("Nicks Error - AppInsights!");
var telemetryClient = Host.Services.GetRequiredService<TelemetryClient>();
telemetryClient.TrackEvent("Test event");
Here I make the distinction between a trace and an event because Application Insights sees these as different things. If you click on the Events node under Usage, you can see information about entries made by calling TrackEvent.
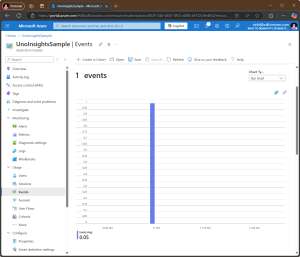
Alternatively, if you click on the Logs node under Monitoring, you can invoke a query to see all the traces. This includes entries made using logging in the application – be aware that any filtering specified in the logBuilder configuration is done in the application before entries are sent to Application Insights.
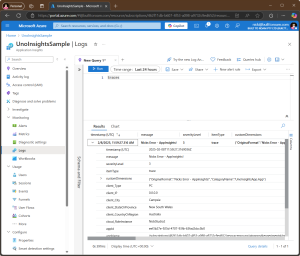
Ok, there you have it, a quick summary of how you can extend logging in your Windows and cross platform application using Azure Application Insights.