I’ve touched on implicit and explicit styles a couple of times in previous posts (eg here and here) when talking about XAML styles. In this post I wanted to provide a more detailed explanation on what implicit and explict styles are and how they should be used in XAML to style controls. In discussing style I’m going to do it in the context of a WinUI/WinAppSdk application but the same principles apply if you’re building a WPF, UWP or Uno application.
Let’s start off by defining what a Style is:
Contains property setters that can be shared between instances of a type. A Style is usually declared in a resources collection so that it can be shared and used for applying control templates and other styles.
Microsoft documentation
With this in mind, let’s create a Style for a Button that sets the Foregound color of the Button to Blue. Here’s what our standard button looks like before we apply a Style.
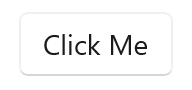
Explicit Style
The Style for the Button has a single Setter that changes the Foreground of the Button:
<Style x:Key="ForegroundButtonStyle"
TargetType="Button">
<Setter Property="Foreground"
Value="Blue" />
</Style>
We can apply this Style to the Button by setting the Style attribute:
<Button Style="{StaticResource ForegroundButtonStyle}">Click Me</Button>
And now the Button looks like this
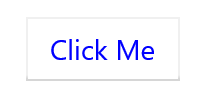
What we have created is an Explicit Style since it only applies to Button elements where the Style property has been explicitly set to the ForegroundButtonStyle.
Implicit Style
Now, let’s say we want our ForegroundButtonStyle to apply to all Buttons in our application. The first option is that we can simply remember to set the Style property on each Button. A better alternative would be to define an Implicit Style, a style that is implied on all Buttons in the application.
All we have to do to change our explicit Style into an explicit Style is to remove the x:Key attribute set on the Style resource:
<Style TargetType="Button">
<Setter Property="Foreground"
Value="Blue" />
</Style>
Since we no longer have a ForegroundButtonStyle we need to remove the Style property off any Button elements that are referencing the ForegroundButtonStyle. All the Button elements (assuming they don’t have a Style attribute set) will now use this implicit Style.
Combining Implicit and Explicit
We have another Button in our application where we want to adjust the corner radius of the Button, so we can again create and use an explicit Style.
<Style x:Key="RoundedButtonStyle"
TargetType="Button">
<Setter Property="CornerRadius"
Value="30" />
</Style>
<Button Style="{StaticResource RoundedButtonStyle}">Click Me</Button>
And our Button now looks like this
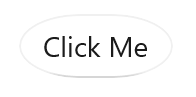
But wait, what happened to our implicit Style – why isn’t the Foreground on the Button blue? By explicitly setting the Style attribute on the Button, the implicit Style is no longer being applied.
Luckily, we can create derived styles using the BasedOn attribute eg BasedOn="{StaticResource MyBaseStyle}"
but here’s the issue, we can’t reference our implicit Style because it doesn’t have a x:Key defined.
This leads us to what I recommend which is to always define both an explicit and implicit style whenever you’re defining an implicit style. By this I mean, create an explicit Style which sets the various properties you want to define in your implicit Style. Next, create an implicit Style that is BasedOn the explicit Style you just created. In our example it would look like this.
<!-- Define the explicit Style that defines all the setters -->
<Style x:Key="MyDefaultButtonStyle"
TargetType="Button"
BasedOn="{StaticResource DefaultButtonStyle}">
<Setter Property="Foreground"
Value="Blue" />
</Style>
<!-- Define the implicit Style BasedOn the explicit Style -->
<Style TargetType="Button"
BasedOn="{StaticResource MyDefaultButtonStyle}" />
<!-- Other explicit Styles can be BasedOn the explicit Style too -->
<Style x:Key="RoundedButtonStyle"
TargetType="Button"
BasedOn="{StaticResource MyDefaultButtonStyle}">
<Setter Property="CornerRadius"
Value="30" />
</Style>
And now our Buttons look like:
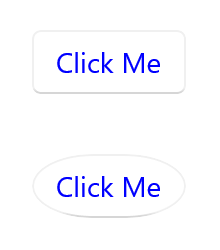
One thing you’ll also note from the explicit MyDefaultButtonStyle that we defined is that it too has a BasedOn attribute set. In this case it is BasedOn the DefaultButtonStyle which (as I discuss in this post) is the explicit Style used by WinUI as the BasedOn for the implicit Style – in other words, MyDefaultButtonStyle is inheriting all the default properties from the implicit WinUI Button Style.
Implicit ResourceDictionary Keys
If you’re familiar with XAML resource dictionaries (ie where you define Styles, Templates and other resources for your app) you’ll know that every resource you define has to have an x:Key defined. The x:Key is what’s used to locate a resource either in XAML (eg Style="{StaticResource MyStyle}"
) or in code (eg App.Current.Resources["MyStyle"]
).
So you might be wondering why we were able to define an Implicit Style that didn’t have a x:Key attribute set. The reason for this is that Styles are a little different from other resources in that in the absence of an x:Key attribute, the TargetType is used as the key.
If we look at the list of resources defined for our application, you can see that we have the MyDefaultButtonStyle and RoundedButtonStyle in the list of keys. We also have the Button Type as a key, this is the registered key for our implicit Button Style.
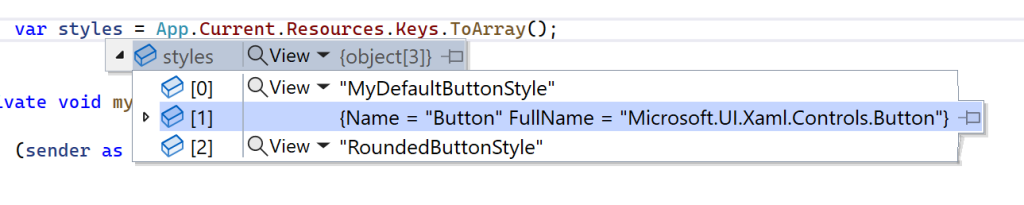
Hopefully this helps clear up what implict and explicit Styles are and how you can use them effectively in your XAML based application.
1 thought on “Implicit and Explicit Styles in XAML”
Comments are closed.