In the previous post I walked through creating an application using MVVM and the MVUX (developed by the Uno Platform) to give an overview comparison of the two frameworks. In this post, I’m going to explain a bit more about MVUX and I’m going to use MVVM as a point of reference.
Model-View-ViewModel (MVVM)
Let’s get started by doing a bit of a refresher on what is Model-View-ViewModel (MVVM). There are plenty of different definitions for what MVVM is and how it should be applied but in essence it relies on the use of data binding to connect the View (typically, but not always, done in XAML) and ViewModel.
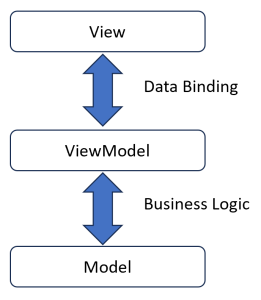
You can think of the ViewModel being the current state of the View. As properties in the ViewModel are set, the ViewModel raises a PropertyChanged event (from the INotifyPropertyChanged interface) and the View queries the property in order to update any element that has been data bound to the property. Actions from the user typically fall into two buckets that either cause a property on the ViewModel to be updated (two-way data binding, for example on a TextBox) or for a method to be executed (typically via a Command that is data bound to an element, for example the Command property on a Button).
Beyond the ViewModel, the definition of the Model and how the ViewModel interacts with the Model isn’t well defined. Depending on what framework you’re using (eg Prism, MvvmCross, Uno.Extensions.Navigation, or a custom implementation) the ViewModel might be injected with any services it needs in order to fetch/update data. Alternatively, in some cases the ViewModel might interact directly with storage or web services to get/set data (ie the Model).
This lack of definition of the Model and the mutability of the ViewModel is where this architecture often falls over. There’s a lot of boilerplate code that a developer has to write in order to get even basic functionality to work (as shown in my previous post where additional properties were added to control visual states of the page). Furthermore, because the logic for fetching and updating data needs to be done asynchronously (to prevent blocking the UI), this can lead to threading issues.
Model-View-Update-eXtended (MVUX)
The basic premise of Model-View-Update is the unidirectional flow and immutability of data. The Model is immutable and any updates yield a new Model. The View is a transform of the Model, so when a new Model is created, the View is rebuilt.
In the world of XAML and data binding, recreating the View with each change to the Model is inefficient and would cause nasty visual artifacts. As such, the various attempts at implementing MVU for XAML typically rely on a caching/differencing layer that ensures only the part of the View that has changed is updated. In effect, they’re rebuilding all the capabilities that come free in the box with data binding.
Model-View-Update-eXtended (MVUX) looks to take advantage of all the power of XAML and data binding, and yet still offer an immutable approach to defining the Model and applying updates. At the heart of this system is the notion of a feed, which in many regards is not too dissimilar to an Observable from Reactive. However, a feed exposes more information than simply a stream of data; it contains information about the progress of the feed, such as when it’s in progress, when it’s got data, when there’s an error and when there’s no data.
The following diagram helps to describe how MVUX works. Similar to MVU you can see that there is a unidirectional flow of data with updates creating new instances of the Model.
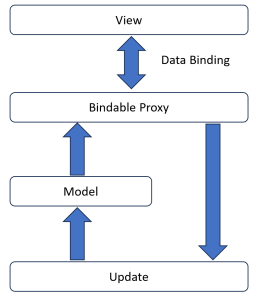
The big difference is that there is a layer of bindable proxies that are source code generated to allow the Model to be data bound to the View. When constructing the View, you still use the same data binding expressions you’re familiar with, and methods on the Model are exposed as command, allowing them to be data bound to elements.
As we saw in the previous post the FeedView control can be used to take advantage of the additional information exposed by a feed. This greatly simplifies both the Model (ie no extra properties required to express the state of data loading) and the View (no need to define visual states).
In this post I’ve provided a high level comparison of the architecture of both MVVM and MVUX but this really just stratches the surface regarding MVUX. The best way to get familiar with MVUX is to give it a shot – use the Uno Platform Wizard in Visual Studio and select the Default configuration which uses MVUX. Then check out the documentation for more information on feeds, states and the FeedView control.
2 thoughts on “MVVM versus MVUX”