In my previous post I covered Dependency Injection for Windows and Uno Platform Applications which covered creating a new application and adding dependency injection using the Uno.Extensions which leverages the Microsoft Extensions IHostBuilder to create an IHost instance. In this post we’re going to adjust the IHostBuilder to setup Logging support.
We’re going to start where the previous post left off with the OnLaunched method:
protected async override void OnLaunched(LaunchActivatedEventArgs args)
{
var builder = this.CreateBuilder(args)
.Configure(host => host
#if DEBUG
// Switch to Development environment when running in DEBUG
.UseEnvironment(Environments.Development)
#endif
);
Host = builder.Build();
var env = Host.Services.GetService<IHostEnvironment>();
MainWindow = builder.Window;
var frame = new Frame();
MainWindow.Content = frame;
frame.Navigate(typeof(MainPage));
MainWindow.Activate();
}
Adding logging is simple as adding a call to UseLogging()
to the IHostBuilder
.
var builder = this.CreateBuilder(args)
.Configure(host => host
...
.UseLogging()
);
Note: If you’re adding this logic to an existing Uno Platform application, make sure you add a reference to Uno.Extensions.Logging.WinUI the the main project of your application.
The UseLogging
method will configure the IHost instance for logging. This will setup both the log output, for example the Output window in Visual Studio and the console, as well as configuring the IServiceProvider
instance (the DI container) will return ILogger
instances when requested.
var logger = Host.Services.GetRequiredService<ILogger<App>>();
logger.LogInformation("Information message");
logger.LogError("Error message");
When the application is run, in this case the Windows target, the log output is displayed in the Output window in Visual Studio.
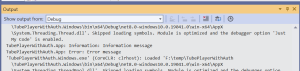
For WASM applications, the log output is also displayed in the browser console. On Edge & Chrome from F12 to open the development tools window and switch to the Console.
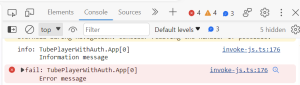
If you want to filter the output in the Console you can change the Log Level dropdown.
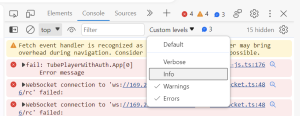
For the GTK target the log messages will appear in the console window, which will appear when running the application in debug (the OutputType is set to Exe)
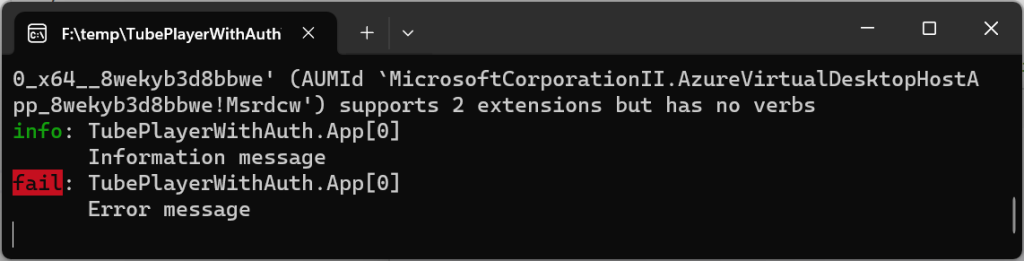
For iOS and Android applications, the log output should appear in the Output window. For Android, you can also access logs via the Device Log.
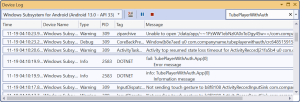
Sometimes it’s desirable to adjust the amount of log messages that are written to the output. For this you can set the minimum log level. In particular, it’s often necessary to display a different level of output when debugging the application from when it’s running in production. In the following code, the minimum level is set to Warning when the hosting environment is set to development, otherwise Error.
.UseLogging(configure: (context, logBuilder) =>
{
// Configure log levels for different categories of logging
logBuilder
.SetMinimumLevel(
context.HostingEnvironment.IsDevelopment() ?
LogLevel.Warning :
LogLevel.Error);
})
It’s also possible to route the internal log messages from Uno into the same output sinks as the main application. This is particularly useful if you’re debugging an issue that isn’t directly related to the code of your application. For this, you need to set the enableUnoLogging parameter to true.
.UseLogging(configure: (context, logBuilder) =>
{
// Configure log levels for different categories of logging
logBuilder
.SetMinimumLevel(
context.HostingEnvironment.IsDevelopment() ?
LogLevel.Warning :
LogLevel.Error)
// Default filters for core Uno Platform namespaces
.CoreLogLevel(LogLevel.Trace);
}, enableUnoLogging: true)
The CoreLogLevel sets the log filter level for namespaces Uno, Microsoft and Windows. You can set additional namespace filters using the AddFilter
method.
In this post we’ve covered adding logging to an application. If you want additional options for configuring the log output you can use the Uno.Extensions.Logging.Serilog package which adds support for Serilog logging. In the coming posts will continue with adding configuration, http and other features to the sample app.
Great article. I was just reading over it to get some inspiration for some improved documentation I’m writing. I found there’s an invalid link in the post to the Uno.Extensions.Logging.WinUI package on NuGet. I think there’s an ‘I’ missing at the end of the URL.