In my previous two posts (here and here) I’ve talked about the new wizard that’s available for creating multi-platform applications with .NET and the Windows App SDK (WinUI), leveraging the Uno Platform. Now we’re going to jump in and take a look at the options that are available via the Customize button. In this post we’ll look at the first three sections: Framework, Platforms and Presentation.
Framework
The first section that’s available for customizing the Uno Platform solution template is to set the .NET framework version. This option defaults to .NET 7 as this is the latest stable release.
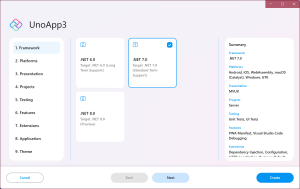
The wizard includes .NET 6 support for anyone wanting to stick with a long term support (LTS) release. Mobile support (iOS, Android and MacCatalyst) was only added, by Microsoft, to .NET late in the .NET 6 cycle, along with the release of .NET MAUI (previously it was Xamarin for iOS/Android etc). As such the tooling and stability was still in-flux. This has been significantly improved in .NET 7, which is why this is the default option.
If you’re curious about the future, feel free to check out the .NET 8 support but as this is in preview, it’s likely you’ll see some issues/instability. You’ll also need to make sure you install the .NET 8 SDK in order to get things to build and run.
If you decide to upgrade (from .NET 6 to .NET 7, or from .NET 7 to .NET 8) or downgrade, after you’ve created your application you can simply change the TargetFrameworks property in each project file (csproj), replacing netX.x with netY.y (eg changing net6.0 to net7.0). Note that you’ll be prompted by Visual Studio to reload projects, which you should do, and you’ll need to force a rebuild (and perhaps even restart Visual Studio) in order for everything to build successfully after changing the framework version.
Platforms
Of all the .NET multi-platform frameworks, the Uno Platform supports the widest range of target platforms. By default, iOS, Android, Web (WebAssembly/WASM), MacCatalyst, Windows and GTK (linux) are selected but there’s also support for Linux (framebuffer) and WPF (skia rendering).
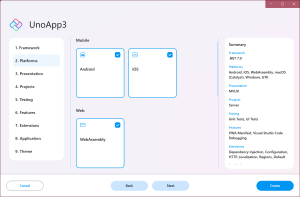
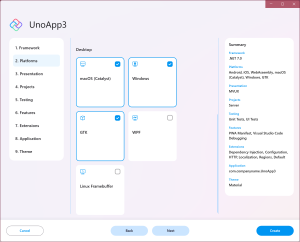
You can select as many or as few platforms as you like, and don’t worry, you can easily add or remove platforms later.
Presentation
The last section we’re going to cover here is the presentation technology. If you’ve worked with XAML you’d be familiar with MVVM. However, this heavily relies on having a mutable view model which propagates changes to the view via data binding. State needs to be explicitly managed within the view model by the developer.
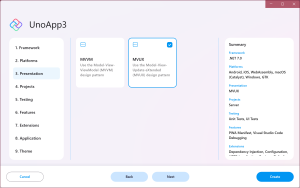
The Uno Platform offers an alternative pattern, Model-View-Update-eXtended (MVUX). Instead of mutable view models, this pattern uses immutable models and a combination of IFeed and IState properties, as shown in the following code snippet.
public partial record MainModel
{
public string? Title { get; }
public IState<string> Name { get; }
public MainModel(
INavigator navigator,
IStringLocalizer localizer)
{
_navigator = navigator;
Title = $"Main - {localizer["ApplicationName"]}";
Name = State<string>.Value(this, () => string.Empty);
}
public async Task GoToSecond()
{
var name = await Name;
await _navigator.NavigateViewModelAsync<SecondModel>(this, data: new Entity(name!));
}
private INavigator _navigator;
}
MVUX is a topic that requires a deep dive in its own right, so if you’re curious, check out the documentation here. We would love feedback on MVUX and in particular any challenges you might run into in getting started with it.
In this post we’ve covered the first three sections in the Uno Platform Template wizard. I’ll be posting more on the other sections in subsequent posts.
I would like to take this approacher, however, my INavigationService is in my portable project and currently call the INavigator form the Uno project as I need the whole navigation take place vm to vm in my portable project.
This ‘Shell’ and/or Uno seem to be generating a lot of c# but I don’t know how and where to start debugging as my Wasm app doesn’t go pass the splash screen.